The CliBuilder
class for quickly and concisely building command line applications has been renewed in Apache Groovy 2.5.
This is the second of a two-part article series that highlights what is new.
In case you missed it, Part 1 is here. This article shows some of the advanced features of the underlying libraries from CliBuilder.
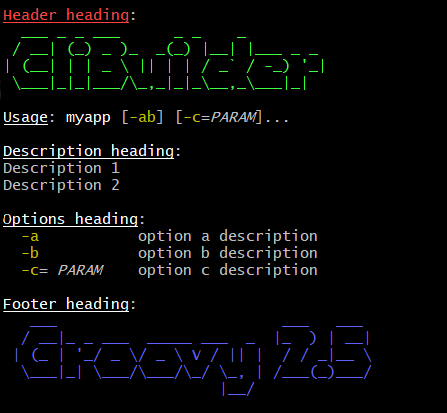
A quick recap of part 1: The groovy.util.CliBuilder
class is deprecated. Instead there are now two CliBuilder implementations in different modules, one with Apache Commons CLI as the underlying parser library, and a new one based on the picocli parser.
It is recommended that applications explicitly import either groovy.cli.picocli.CliBuilder
or groovy.cli.commons.CliBuilder
.
The groovy.util.CliBuilder
class is deprecated and delegates to the Commons CLI version for backwards compatibility.
New features will likely only be added to the picocli version, and groovy.util.CliBuilder
may be removed in a future version of Groovy. The Commons CLI version is intended for applications that rely on the internals of the Commons CLI implementation of CliBuilder and cannot easily migrate to the picocli version.
Next, let’s take a look at some advanced features offered by these underlying command line parsing libraries.
Apache Commons CLI Features
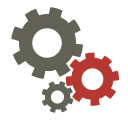
Sometimes you may want to use advanced features of the underlying parsing library.
For example, you may have a command line application with mutually exclusive options.
The below code shows how to achieve this using the Apache Commons CLI OptionGroup
API:
import groovy.cli.commons.CliBuilder
import org.apache.commons.cli.*
def cli = new CliBuilder()
def optionGroup = new OptionGroup()
optionGroup.with {
addOption cli.option('s', [longOpt: 'silent'], 's option')
addOption cli.option('v', [longOpt: 'verbose'], 'v option')
}
cli.options.addOptionGroup optionGroup
assert !cli.parse('--silent --verbose'.split()) (1)
1 | Parsing this input will fail because two mutually exclusive options were specified. |
Picocli CliBuilder Features
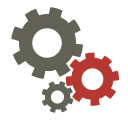
Strongly Typed Lists
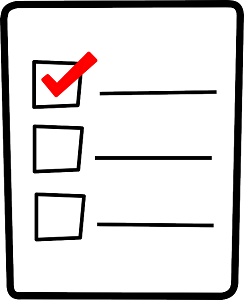
Options with multiple values often use an array or a List to capture the values.
Arrays can be strongly typed, that is, contain elements other than String.
The picocli version of CliBuilder lets you do the same with Lists.
The auxiliaryType
specifies the type that the elements should be converted to.
For example:
import groovy.cli.picocli.CliBuilder
def cli = new CliBuilder()
cli.T(type: List, auxiliaryTypes: Long, 'typed list') (1)
def options = cli.parse('-T 1 -T 2 -T 3'.split()) (2)
assert options.Ts == [ 1L, 2L, 3L ] (3)
1 | Define an option that can have multiple integer values. |
2 | An example command line. |
3 | The option values as a List<Integer> . |
Strongly Typed Maps
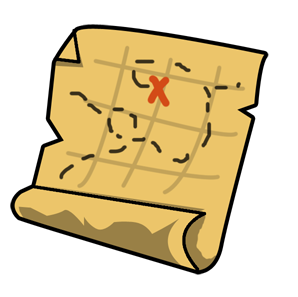
The picocli version of CliBuilder offers native support for Map options.
This is as simple as specifying Map as the option type.
By default, both keys and values are stored as Strings in the Map,
but it’s possible to use auxiliaryType
to specify the types that the keys and values should be converted to.
import groovy.cli.picocli.CliBuilder
def cli = new CliBuilder()
cli.D(args: 2, valueSeparator: '=', 'Commons CLI style map') (1)
cli.X(type: Map, 'picocli style map support') (2)
cli.Z(type: Map, auxiliaryTypes: [TimeUnit, Integer].toArray(), 'typed map') (3)
def options = cli.parse('-Da=b -Dc=d -Xx=y -Xi=j -ZDAYS=2 -ZHOURS=23'.split()) (4)
assert options.Ds == ['a', 'b', 'c', 'd'] (5)
assert options.Xs == [ 'x':'y', 'i':'j' ] (6)
assert options.Zs == [ (DAYS as TimeUnit):2, (HOURS as TimeUnit):23 ] (7)
1 | Commons CLI has map-like options by specifying that each option must have two parameters, with some separator. |
2 | The picocli version of CliBuilder has native support for Map options. |
3 | The key type and value type can be specified for strongly-typed maps. |
4 | An example command line. |
5 | The Commons CLI style option gives a list of [key, value, key, value, …] objects. |
6 | The picocli style option gives the result as a Map<String, String> . |
7 | When auxiliaryTypes are specified, the keys and values of the map are converted to the specified types, giving you a Map<TimeUnit, Integer> . |
Usage Help with Detailed Synopsis
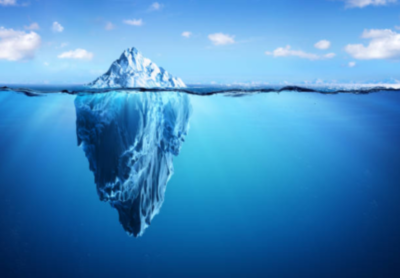
CliBuilder has always supported a usage
property to display the usage help synopsis of a command:
// the old way
new CliBuilder(usage: 'myapp [options]').usage()
The above program prints:
Usage: myapp [options]
This still works, but the picocli version has a better alternative with the name
property.
If you specify name
instead of usage
, picocli will show all options in a succinct synopsis with square brackets [
and ]
for optional elements and ellipsis …
for elements that can be repeated one or more times. For example:
// the new way
def cli = new CliBuilder(name: 'myapp') // detailed synopsis
cli.a('option a description')
cli.b('option b description')
cli.c(type: List, 'option c description')
cli.usage()
The above program prints:
Usage: myapp [-ab] [-c=PARAM]... -a option a description -b option b description -c= PARAM option c description
Use Any Option Names
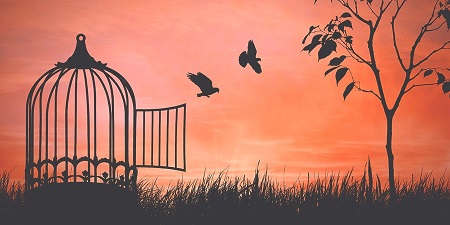
Image credit: (c) PsychoShadow - www.bigstockphoto.com
Before, if an option had multiple names with a single hyphen, you had no choice but to declare the option multiple times:
// before: split -cp, -classpath into two options
def cli = new CliBuilder(usage: 'groovyConsole [options] [filename]')
cli.classpath('Where to find the class files')
cli.cp(longOpt: 'classpath', 'Aliases for '-classpath')
The picocli version of CliBuilder supports a names
property that can have any number of option names that can take any prefix. For example:
// after: an option can have many names with any prefix
def cli = new CliBuilder(usage: 'groovyConsole [options] [filename]')
cli._(names: ['-cp', '-classpath', '--classpath'], 'Where to find the class files')
Fine-grained Usage Help Message
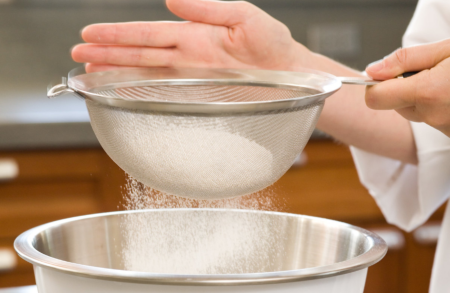
Picocli offers fine-grained control over the usage help message format and this functionality is exposed via the usageMessage
CliBuilder property.
The usage message has a number of sections: header, synopsis, description, parameters, options and finally the footer. Each section has a heading, that precedes the first line of its section. For example:
import groovy.cli.picocli.CliBuilder
def cli = new CliBuilder()
cli.name = "groovy clidemo"
cli.usageMessage.with { (1)
headerHeading("Header heading:%n") (2)
header("header 1", "header 2") (3)
synopsisHeading("%nUSAGE: ")
descriptionHeading("%nDescription heading:%n")
description("description 1", "description 2")
optionListHeading("%nOPTIONS:%n")
footerHeading("%nFooter heading:%n")
footer("footer 1", "footer 2")
}
cli.a(longOpt: 'aaa', 'a-arg') (4)
cli.b(longOpt: 'bbb', 'b-arg')
cli.usage()
1 | Use the usageMessage CliBuilder property to customize the usage help message. |
2 | Headings can contain string format specifiers like the %n newline. |
3 | Sections are multi-line: each string will be rendered on a separate line. |
4 | Define some options. |
This prints the following output:
Header heading: header 1 header 2 USAGE: groovy clidemo [-ab] Description heading: description 1 description 2 OPTIONS: -a, --aaa a-arg -b, --bbb b-arg Footer heading: footer 1 footer 2
Usage Help with ANSI Colors
Out of the box, the command name, option names and parameter labels in the usage help message are rendered with ANSI styles and colors. The color scheme for these elements can be configured with system properties.
Other than that, you can use colors and styles in the descriptions and other sections of the usage help message, using a simple markup notation. The example below demonstrates:
def cli = new groovy.cli.picocli.CliBuilder(name: 'myapp')
cli.usageMessage.with {
headerHeading("@|bold,red,underline Header heading|@:%n")
header($/@|bold,green \
___ _ _ ___ _ _ _
/ __| (_) _ )_ _(_) |__| |___ _ _
| (__| | | _ \ || | | / _` / -_) '_|
\___|_|_|___/\_,_|_|_\__,_\___|_|
|@/$)
synopsisHeading("@|bold,underline Usage|@: ")
descriptionHeading("%n@|bold,underline Description heading|@:%n")
description("Description 1", "Description 2") // after the synopsis
optionListHeading("%n@|bold,underline Options heading|@:%n")
footerHeading("%n@|bold,underline Footer heading|@:%n")
footer($/@|bold,blue \
___ ___ ___
/ __|_ _ ___ _____ ___ _ |_ ) | __|
| (_ | '_/ _ \/ _ \ V / || | / / _|__ \
\___|_| \___/\___/\_/ \_, | /___(_)___/
|__/ |@/$)
}
cli.a('option a description')
cli.b('option b description')
cli.c(type: List, 'option c description')
cli.usage()
The code above gives the following output:
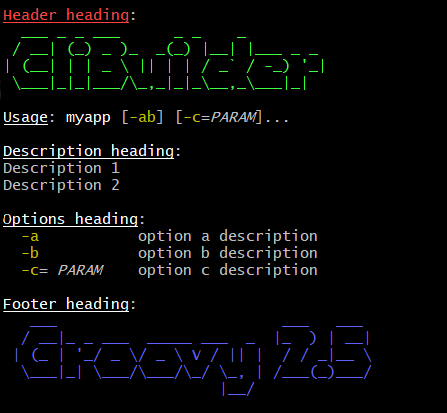
(Credit to http://patorjk.com/software/taag/ for the ASCII art.)
New errorWriter
Property
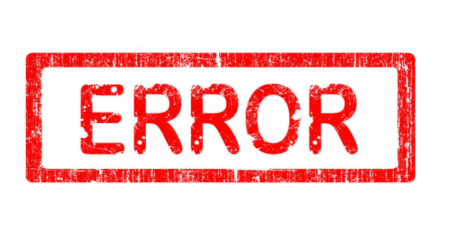
When the user provided invalid input, the picocli version of CliBuilder writes an error message and the usage help message to the new errorWriter
property (set to System.err
by default).
When the user requests help, and the application calls CliBuilder.usage()
, the usage help message is printed to the writer
property (System.out
by default).
Previous versions of CliBuilder used the writer
property for both invalid input and user-requested help.
Why this change? This helps command line application authors to follow standard practice and separate diagnostic output from the program output: If the output of a Groovy program is piped to another program,
sending error messages to STDERR prevents the downstream program from inadvertently trying to parse error output.
On the other hand, when users request help with --help
or --version
, the output should be sent to STDOUT,
because the user may want to pipe the output to a utility like less
or grep
.
For backwards compatibility, setting the writer
property to another value will also set the errorWriter
to the same value.
(You can still set the errorWriter
to another value afterwards if desired.)
Conclusion
Groovy 2.5 CliBuilder offers a host of exciting new features. Try it out and let us know what you think!
This is part 2 of a two-part article. In case you missed it, here is Part 1.
For more information, visit the Groovy site and GitHub project, and the picocli site and picocli GitHub project. Please star the projects if you like what you see! |